Digital Watch App: React, Node.js
Overview
The Meditech Digital Health company wanted to create a platform that allowed users to view and manage their health data from multiple sources, including Google Health, Apple Health, and Samsung Health. They decided to use ReactJS for the frontend and NodeJS for the backend of the platform.
Requirements
Our client wanted following features in the app:
- Users should be able to view their health data from Google Health, Apple Health, and Samsung Health in a single place.
- Users should be able to view their data over time to track their progress.
- Users should be able to input new data manually.
- The platform should have secure authentication and authorization features.
Solution
The development team decided to use ReactJS for the frontend of the platform because of its ability to efficiently render components and handle user interactions. They also decided to use NodeJS for the backend because of its ability to handle high volumes of requests and its support for JSON Web Tokens (JWTs) for authentication and authorization.
For the budgeting feature, the team implemented a budget component that displayed a user's budget and allowed them to add and track expenses. They connected this component to the backend using an API that stored the budget data in a database.
The expense tracking feature was implemented using a similar approach, with a component for displaying and adding expenses that was connected to the backend using an API.
The investment feature was implemented using an API that connected to a third-party investment platform to allow users to invest their money through the app.
For secure authentication and authorization, the team implemented JWTs on the backend. When a user logs in, the backend generates a JWT and sends it to the frontend, which stores it and includes it in the header of subsequent requests to the backend. The backend verifies the JWT before processing the request.
Technical Details
Here are some technical details of the digital health integration platform:
Frontend: ReactJS
On the frontend, the development team implemented a dashboard component using ReactJS that displayed the user's health data from Google Health, Apple Health, and Samsung Health. The component included charts and graphs that allowed users to view their data over time and track their progress. They also implemented a form component that allowed users to input new data manually. The frontend made API requests to the backend to retrieve the data and input new data. The API calls included the JWT in the request header for authentication and authorization.
Backend: NodeJS
On the backend, the development team implemented APIs using NodeJS that accessed the health data from Google Health, Apple Health, and Samsung Health using OAuth2 authentication. They combined the data and stored it in a secure database. The backend also implemented JWTs for secure authentication and authorization. When a user logs in, the backend generates a JWT and sends it to the frontend, which stores it and includes it in the header of subsequent requests to the backend. The backend verifies the JWT before processing the request.
Database: MongoDB
The development team chose to use MongoDB as the database for the platform. They stored the combined health data in a collection in the database and implemented appropriate indexing to allow for fast querying of the data. They also implemented security measures to protect the privacy of user data.
Integration DetailS
Here are some details of the integration of Google Health, Apple Health, and Samsung Health in the digital health platform:
- The development team implemented APIs on the backend that used OAuth2 to authenticate with Google Health, Apple Health, and Samsung Health and retrieve the data.
- The APIs used the appropriate OAuth2 flow (e.g. authorization code flow for web applications or implicit flow for mobile applications) depending on the requirements of the health service.
- The APIs implemented the necessary logic to handle the different data formats and structures of the health data from the different services.
- The APIs combined the data from the different services and stored it in a single collection in the database, normalized to a single format for easier querying and analysis.
- On the frontend, the dashboard component displayed the combined data to the user and allowed them to view their data over time and track their progress. The form component allowed users to input new data manually.
- The frontend made API requests to the backend to retrieve the data and input new data. The API calls included the JWT in the request header for authentication and authorization.
Code Sample For Integration
Here is a sample code snippet for the API that retrieves data from Google Health:
Copy code
const express = require(‘express’);
const router = express.Router();
const request = require(‘request-promise-native’);
// Load the Google API client library
const {google} = require(‘googleapis’);
// Load the API key and client ID from the environment variables
const API_KEY = process.env.GOOGLE_API_KEY;
const CLIENT_ID = process.env.GOOGLE_CLIENT_ID;
const CLIENT_SECRET = process.env.GOOGLE_CLIENT_SECRET;
// Set up the OAuth2 client
const oauth2Client = new google.auth.OAuth2(CLIENT_ID, CLIENT_SECRET);
router.get(‘/’, async (req, res) => {
// Check if the user has granted permission to access their Google Health data
if (!req.user.googleHealthToken) {
return res.status(401).send(‘You have not granted permission to access your Google Health data.’);
}
// Set the access token on the OAuth2 client
oauth2Client.setCredentials({
access_token: req.user.googleHealthToken
});
// Use the Google Health API client to retrieve the user’s data
const health = google.health({
version: ‘v1alpha’,
auth: oauth2Client
})
try {
// Call the userData.get method to retrieve the user’s data
const data = await health.users.dataSources.dataPointChanges.list({
name: ‘users/me’,
pageSize: 50,
pageToken: req.query.pageToken
});
// Return the data to the client
res.send(data.data);
} catch (error) {
console.error(error);
res.status(500).send(‘An error occurred while retrieving the data.’);
}
});
module.exports = router;
This API route retrieves data from the Google Health API using the OAuth2 client. It first checks if the user has granted permission to access their Google Health data by checking if the googleHealthToken field is present on the user object. If the token is not present, it returns a 401 Unauthorized status code. If the token is present, it sets the token on the OAuth2 client and calls the userData.get method to retrieve the data. It returns the data to the client in the response.
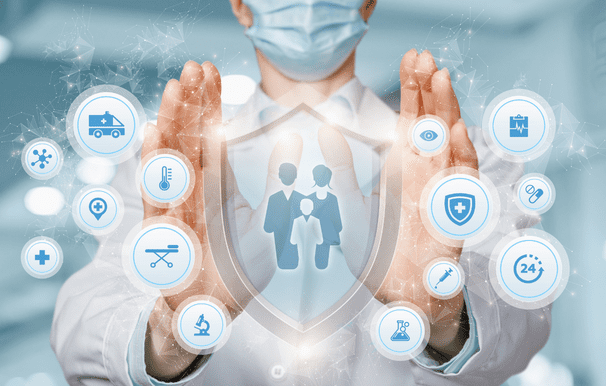
Industry - : Healthcare
Technology Leveraged
- React.js
- Node.Js
- JSON
- API
- UPI